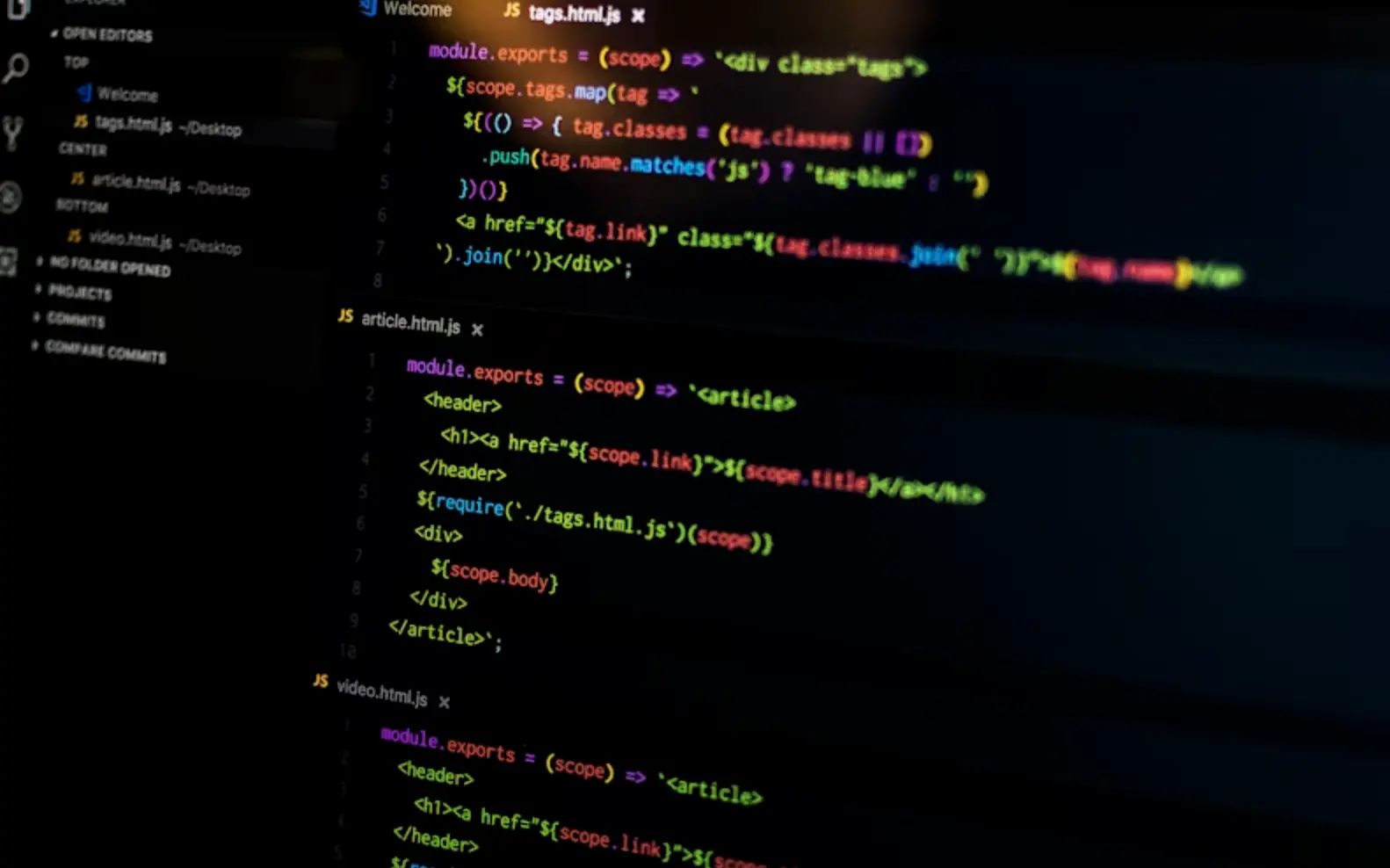
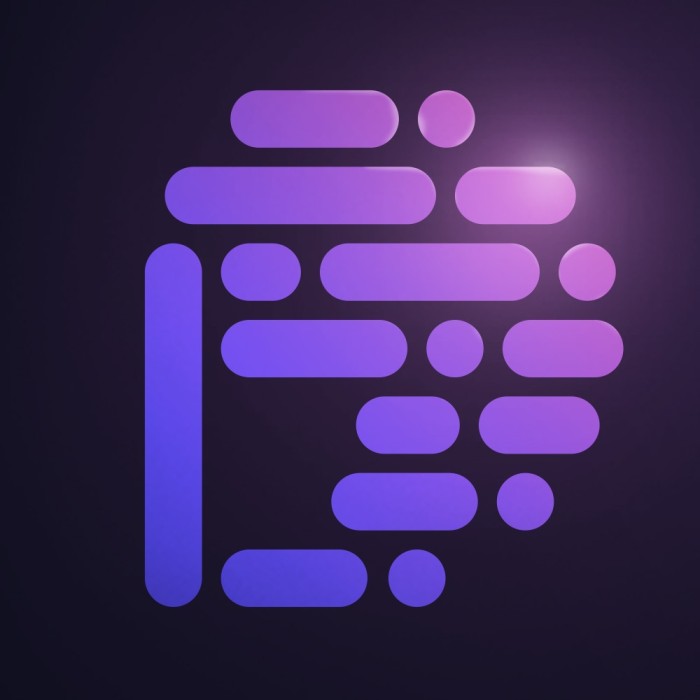
bongoDev
TypeScript Best Practices for Large Scale Applications - bongoDev
Why TypeScript is Essential for Large-Scale Applications
As applications grow in complexity, managing code efficiently becomes a challenge. TypeScript helps developers write safer, more maintainable code, making it an essential tool for large-scale projects. This blog covers best practices for using TypeScript effectively.
1. Mastering TypeScript's Type System
TypeScript’s powerful type system helps prevent runtime errors and improves code clarity. Here are key best practices:
- Advanced Type Features: Use union, intersection, and mapped types to handle complex data structures and ensure flexibility in your code.
- Generic Types: Generics help create reusable components by defining types dynamically. This is especially useful in functions and custom hooks.
- Utility Types: TypeScript provides built-in utility types like
Partial<T>
,Pick<T, K>
, andOmit<T, K>
to make type transformations easier. - Type Inference: Instead of explicitly defining every type, leverage TypeScript’s inference system to reduce boilerplate and improve readability.
2. Organizing Large-Scale TypeScript Projects
A well-structured project improves maintainability and scalability. Consider these best practices:
- Module Organization: Keep code modular by grouping related files into separate folders. Use a clear naming convention for files and directories.
- Type Declaration Files: Use
.d.ts
files for external libraries and shared types to maintain consistency across the project. - Configuration Options: Optimize your
tsconfig.json
file with strict mode enabled and appropriate compiler options to enforce best practices. - Build Tooling: Use tools like Webpack, Babel, or esbuild to bundle and optimize TypeScript code efficiently.
3. Effective Testing Strategies in TypeScript
Testing is critical to ensure code reliability and maintainability. Here’s how to approach testing in TypeScript:
- Type Testing: Use tools like
tsd
or@typescript-eslint
to validate types and detect potential issues early. - Unit Testing with Types: Use Jest, Mocha, or Vitest with TypeScript to write type-safe unit tests.
- Integration Testing: Ensure seamless communication between different parts of the application by testing API responses and database interactions.
- Test Organization: Maintain a clear folder structure for tests, following best practices like placing test files next to the implementation or in a dedicated
tests
directory.
TypeScript provides powerful tools for building and maintaining large-scale applications efficiently. By following these best practices, developers can write scalable, maintainable, and error-free code. Stay updated with new TypeScript features and continuously improve your project structure for long-term success.