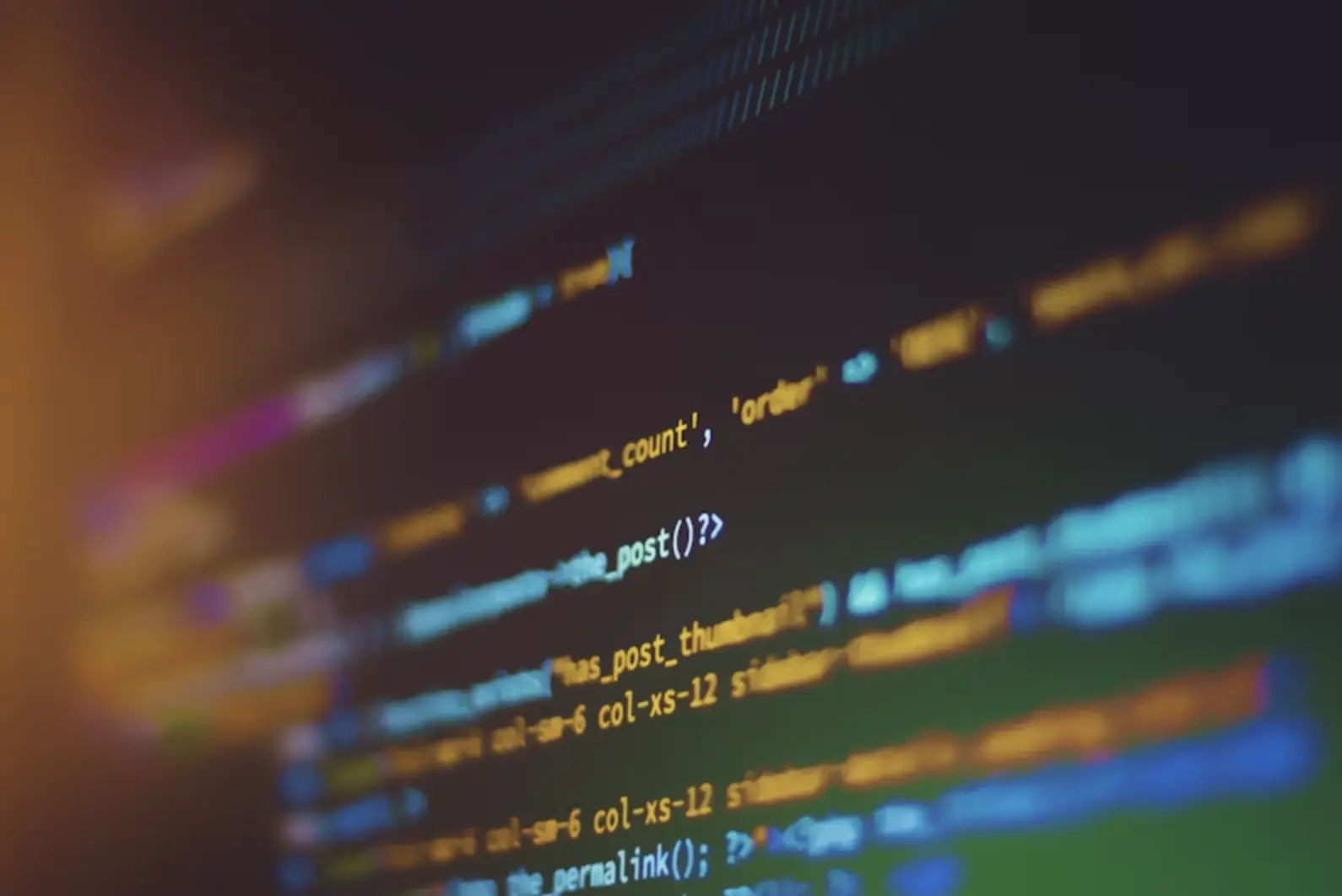
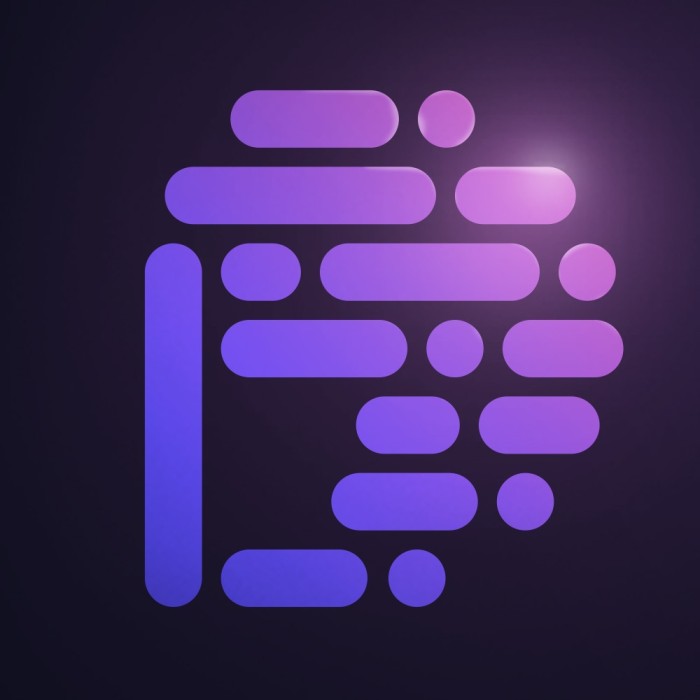
bongoDev
Advanced API Security Patterns and Best Practices - bongoDev
Effective Ways to Secure Modern APIs
As more applications depend on APIs, ensuring proper security is critical. Weak API security can expose sensitive data and make applications vulnerable to attacks. Here are the most important security patterns and best practices you should follow when building modern APIs.
1. Best Practices for API Authentication
Authentication is the process of verifying who the user or system is. Strong authentication ensures only valid users can access your API.
- JWT Implementation: JSON Web Tokens (JWT) are popular for stateless authentication. After login, the server issues a token, and the client includes this token in every request. Always sign and validate the token to ensure it hasn’t been tampered with.
- OAuth 2.0 Flows: For APIs that need to work with third-party systems, OAuth 2.0 is the industry standard. Use the correct flow depending on your use case, such as Authorization Code Flow for web apps or Client Credentials Flow for machine-to-machine APIs.
- Session Management: For some applications, especially internal ones, you may use traditional session-based authentication where the session is stored on the server. Make sure sessions expire and are properly encrypted.
- Multi-factor Authentication (MFA): Adding a second layer of authentication (like an OTP code via SMS or authenticator app) improves the overall security, especially for sensitive API endpoints.
2. Advanced Authorization Strategies
Once a user is authenticated, authorization determines what they are allowed to do. Proper authorization prevents unauthorized access to sensitive data or actions.
- Role-Based Access Control (RBAC): Assign users different roles, like Admin, Manager, or User, and control their permissions based on these roles. This is simple and widely used.
- Permission Systems: For more flexibility, define fine-grained permissions for actions like "create", "read", "update", and "delete" and apply them to each user or role.
- Policy Enforcement: Use tools like Open Policy Agent (OPA) to separate authorization logic from application code. This helps you define and enforce complex rules in a central place.
- Resource Protection: Ensure that users can only access their own data. For example, a user should only see their own order history, not someone else’s. Enforce these checks at the database query or service layer.
3. Essential Security Headers and Defenses
API security doesn’t stop at authentication and authorization. You must also protect your API from common web threats by using proper security headers and input controls.
- CORS Configuration: Control which domains are allowed to interact with your API using Cross-Origin Resource Sharing (CORS). Only trusted domains should be allowed in production.
- Content Security Policy (CSP): Set strict rules about which content (scripts, styles, images) can be loaded. This reduces the risk of cross-site scripting (XSS) attacks.
- Rate Limiting: Limit how many requests a user or IP can make in a given time period. This helps prevent abuse, brute force attacks, and accidental overuse of your API.
- Input Validation: Always validate and sanitize user inputs to prevent SQL injection, command injection, and other input-based attacks. Use libraries that automatically handle input validation for your programming language.
By following these advanced API security patterns and best practices, you can build safer, more reliable APIs for your applications. Whether you are building APIs for public use or internal systems, strong security is a must to protect user data and ensure smooth operations.